How to Optimize SEO with Next.js
Carlos Souza at 2025-03-16
Introduction
Search Engine Optimization (SEO) is crucial for improving your website's visibility on search engines like Google and Bing. A well-optimized site attracts more organic traffic, enhances user experience, and ultimately improves conversion rates. Next.js, a powerful React framework, offers built-in features that make it easier to enhance your website's SEO.
In this article, we’ll explore the best practices for optimizing SEO using Next.js, including Server-Side Rendering (SSR), static site generation (SSG), metadata handling, and structured data.
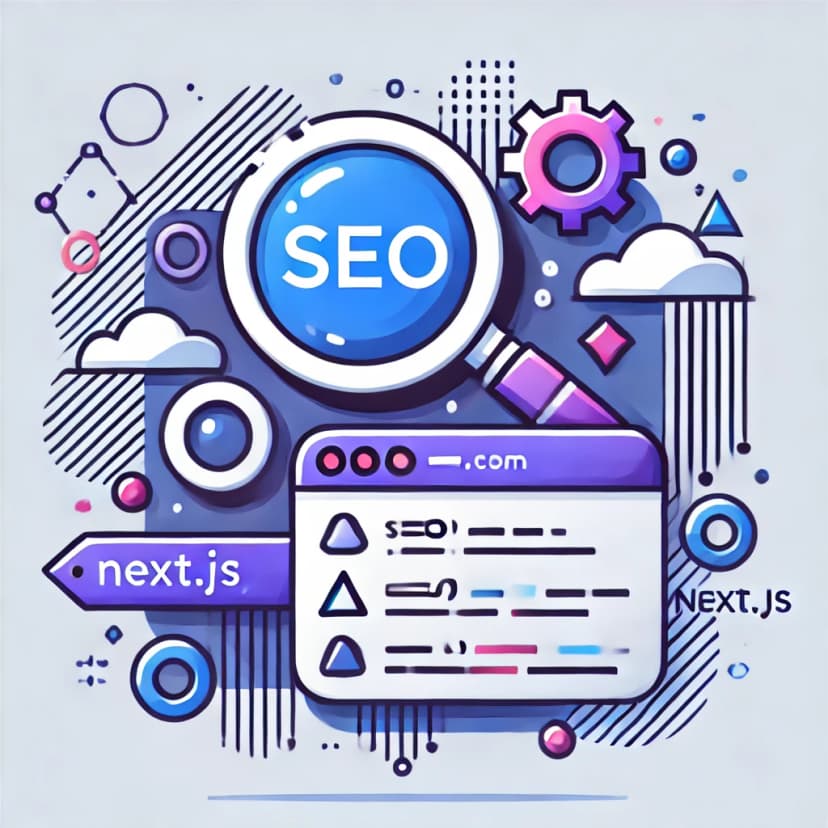
Why Next.js is Great for SEO
Next.js provides several advantages for SEO optimization, including:
- Server-Side Rendering (SSR) – Ensures that content is available to search engines immediately after loading.
- Static Site Generation (SSG) – Improves performance and speeds up indexing.
- Dynamic Routing – Helps to create clean and SEO-friendly URLs.
- Metadata Handling – Simplifies adding meta tags and Open Graph tags for better social media sharing and search ranking.
1. Using Server-Side Rendering (SSR) to Improve SEO
Server-Side Rendering (SSR) allows Next.js to generate content on the server at request time. This makes the content immediately available to search engine crawlers, improving the indexing process.
Example of SSR with Next.js:
export async function getServerSideProps(context) {
const res = await fetch(`https://api.example.com/data`);
const data = await res.json();
return {
props: { data },
};
}
function MyPage({ data }) {
return (
<div>
<h1>{data.title}</h1>
<p>{data.description}</p>
</div>
);
}
export default MyPage;
In this example:
getServerSideProps
fetches data at request time.- The content is fully rendered on the server, which makes it visible to search engines.
- This improves the crawlability and indexing of dynamic content.
2. Implementing Static Site Generation (SSG)
Static Site Generation (SSG) allows Next.js to generate static HTML files at build time. This improves loading speed and SEO performance since the content is already available to search engines.
Example of SSG with Next.js:
export async function getStaticProps() {
const res = await fetch('https://api.example.com/data');
const data = await res.json();
return {
props: { data },
};
}
function MyPage({ data }) {
return (
<div>
<h1>{data.title}</h1>
<p>{data.description}</p>
</div>
);
}
export default MyPage;
SSG Advantages:
- Faster page load times.
- Better Core Web Vitals scores (which are ranking factors).
- Content is immediately indexable by search engines.
3. Configuring sitemap.xml
and robots.txt
A sitemap.xml
file helps search engines understand your site structure and index your pages more effectively. A robots.txt
file allows you to control which pages are indexed.
Example of robots.txt
in Next.js:
Create a file public/robots.txt
:
User-agent: *
Allow: /
Disallow: /private
Example of sitemap.xml
in Next.js:
Create a script scripts/generate-sitemap.js
:
const fs = require('fs');
const pages = [
{ url: '/', priority: 1.0 },
{ url: '/about', priority: 0.8 },
];
const sitemap = `<?xml version="1.0" encoding="UTF-8"?>
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
${pages
.map(
(page) => `
<url>
<loc>${`https://example.com${page.url}`}</loc>
<priority>${page.priority}</priority>
</url>
`
)
.join('')}
</urlset>`;
fs.writeFileSync('public/sitemap.xml', sitemap);
In package.json
, add the script:
"scripts": {
"generate-sitemap": "node scripts/generate-sitemap.js"
}
Best Practices:
- Keep the sitemap updated automatically during build.
- Ensure that all important pages are included.
- Make sure the
robots.txt
file doesn’t block critical pages.
4. Managing Metadata and Open Graph Tags
Metadata is critical for SEO because it helps search engines and social platforms understand your content. Next.js provides a <Head>
component to add meta tags easily.
Example of adding metadata with Next.js:
import Head from 'next/head';
export default function MyPage() {
return (
<>
<Head>
<title>My Next.js Page</title>
<meta name="description" content="This is an example Next.js page" />
<meta property="og:title" content="My Next.js Page" />
<meta property="og:description" content="This is an example Next.js page" />
<meta property="og:url" content="https://example.com" />
<meta property="og:type" content="website" />
</Head>
<div>
<h1>My Next.js Page</h1>
</div>
</>
);
}
5. Adding Structured Data (JSON-LD)
Structured data helps search engines understand the context of your content, which can improve rich search results (e.g., product reviews, star ratings, FAQs).
Example of adding structured data with Next.js:
import Head from 'next/head';
export default function MyPage() {
const structuredData = {
"@context": "https://schema.org",
"@type": "Article",
"headline": "My Next.js Page",
"author": {
"@type": "Person",
"name": "Carlos Souza"
},
"datePublished": "2025-02-19",
"dateModified": "2025-02-19"
};
return (
<>
<Head>
<script
type="application/ld+json"
dangerouslySetInnerHTML={{ __html: JSON.stringify(structuredData) }}
/>
</Head>
<div>
<h1>My Next.js Page</h1>
</div>
</>
);
}
Conclusion
Next.js offers powerful SEO features through Server-Side Rendering (SSR), Static Site Generation (SSG), and advanced metadata handling. By implementing structured data, optimizing page speed, and configuring sitemap and robots.txt files, you can improve your site's search engine rankings and organic traffic.
Related Articles
Improving Next.js Performance
Tips and practices to improve the performance of your Next.js site, ensuring fast loading and a better user experience.